iFrame API lifecycle
API docs for version 1 of the Spreedly iFrame Javascript API.
iFrame overview
For merchants who are not using iframe-v1
, or iframe-stable
, Spreedly has implemented a deprecation plan for iFrame where all versions that are more than 3 months out of date will be deprecated and removed from service. This ensures that all customers are on recent versions with the latest security updates. We strongly recommended implementing one of our regularly updated release channels as they will always be up to date.
Please read the iFrame guide for general-purpose iFrame documentation.
The Spreedly iFrame is available from the following URL:
https://core.spreedly.com/iframe/iframe-v1.min.js
By default, an instance of the Spreedly iFrame will be available on the host page as Spreedly
. Invoke the functions described in these docs using the Spreedly
object.
// Invoke methods on the "Spreedly" global object,
// for instance...
Spreedly.validate();
Lifecycle
Including the iFrame Javascript file on a checkout page does not automatically load the library. The iFrame’s lifecycle must be explicitly managed using the following functions.
Providing all required parameters in every function call is crucial for successful payments and helps to fortify payment pages from bot attacks that test stolen credit card numbers. These steps establish a properly-configured iFrame integration for PCI-compliant card tokenization.
init
Initializes the iFrame library with secure tokenization. This must be the first call made and triggers loading of the iFrame fields.
Signature
init(environmentKey, options)
Spreedly.init("C7cRfNJGODKh4Iu5Ox3PToKjniY", {
"numberEl": "spreedly-number",
"cvvEl": "spreedly-cvv",
"nonce": "dd6b70a5-e070-4fa2-a3ca-0db0b45f78d0", // Unique per session (e.g., UUID)
"timestamp": "1738252535",
"certificateToken": "certificate",
"signature": "SERVER_GENERATED_SIGNATURE" // See our Security Requirements section.
});
The call to
init
must be made after the DOM elements fornumberEl
andcvvEl
have been created.
Security Requirements
Securing the iFrame is critical to minimizing card testing and other automated fraud attempts via botnets. An unsecured iFrame can be exploited as an entry point for malicious scripts that test stolen card details at scale, bypassing frontend protections. Businesses can significantly reduce exposure to automated abuse by implementing robust security measures. This includes authenticating all iFrame requests using secure tokenization described below.
With a secure iFrame as a strong foundation, businesses can further improve protections by configuring proper security headers and applying bot-mitigation techniques such as rate limiting and token validation, among other strategies. These steps can help businesses protect both their customers and their payment pages, with iFrame as a secure component following the steps below:
⚠️ These arguments are required to authenticate requests to Spreedly's API for new and existing payment methods. Further detail on certificates and private key management can be found in our step-by-step guide.
nonce
: A unique, cryptographically random value generated per session to prevent replay attacks.timestamp
: An Epoch-based timestamp after which the request will be considered invalid. 30 minute validity window added so you can use the current time if you don't expect a page session to last longer than 30 minutes.certificateToken
: the Spreedly certificate token returned to a merchant when storing the public portion of their asymmetric key pair; used for verification of the digital signature.signature
: A server-generated hash string created by a merchant using thenonce
+timestamp
+certificateToken
, signed with a merchant-controlled private key.
Arguments
Name | Description |
---|---|
environmentKey | The key of the Spreedly environment where the payment method should be tokenized |
options | Map of initialization options. numberEl should be set to the id of the HTML element where the number iFrame field should be rendered. cvvEl should be set to the id of the HTML element where the CVV iFrame field should be rendered. |
Events
Name | Action |
---|---|
ready | When the iFrame has been initialized, rendered, and is ready for further configuration |
Key Notes
- Security Compliance:
- Enabling this functionality allows for further confidence in risk assessments, performance of rate-limiting and other mitigation strategies for transaction attempts using iFrame on a payment page.
- Signature validation ensures request integrity and authenticity, aligning with PCI-DSS 4.0’s script/data integrity requirements.
- Never hardcode secrets client-side—generate
signature
server-side using a securely stored private key.
- Implementation Steps:
- Generate a unique
nonce
(e.g., UUID) for each payment session, and for each instance of iframe. - Compute
signature
server-side via SHA256 using thenonce
+timestamp
+certificateToken
and your private key. - Send these arguments during the
init
call to ensure authentication of your session and associated Create payment method call.
- Generate a unique
Enabling authentication
To enable authentication of your Create payment method API calls via iFrame, visit the environment settings page in app.spreedly.com.
We recommend selecting iFrame or Express only, and ensuring iFrame is properly configured with the arguments for secure tokenization prior to enabling its setting under "Enhanced Security". Once saved, transactions are authenticated from iFrame in this environment only, using a session nonce + signature and timestamp.
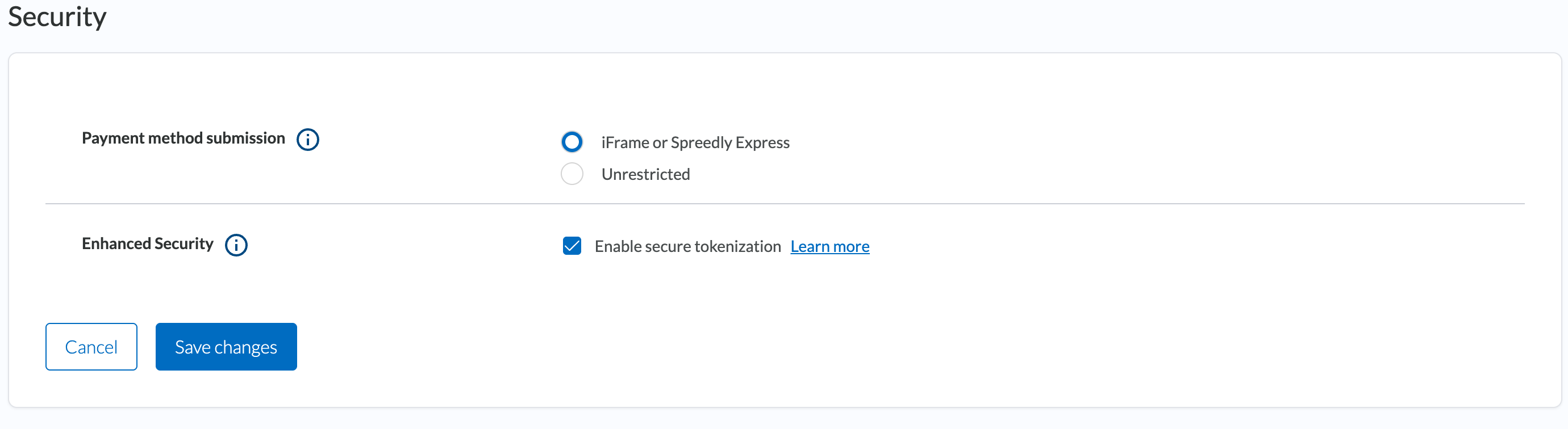
Note: When enabled, all Create payment method calls will be impacted and require authentication. This includes iFrame using the described session nonce authentication mechanism, or basic HTTP authorization for payment methods tokenized via API.
reload
Reload the iFrame library. This resets and re-initializes all iFrame elements and state and is a convenient way to quickly reset the form.
Spreedly.reload();
When reload is complete, the ready
event will be fired, at which time the iFrame can be customized.
Signature
reload()
removeHandlers
Remove all event handlers currently registered via the on
function.
Spreedly.removeHandlers();
Signature
removeHandlers()
SpreedlyPaymentFrame
Create a new, independent, instance of the iFrame. It will be created alongside the default instance, already exposed as Spreedly
. Note that unique security parameters must be passed for this other instance.
// Create a new instance
var otherIFrame = new SpreedlyPaymentFrame();
// Configure as usual
otherIFrame.init("C7cRfNJGODKh4Iu5Ox3PToKjniY", {
"numberEl": "spreedly-number",
"cvvEl": "spreedly-cvv",
"nonce": "dd6b70a5-e070-4fa2-a3ca-0db0b45f78d0", // Unique per session (e.g., UUID)
"timestamp": "1738252535",
"certificateToken": "certificate",
"signature": "SERVER_GENERATED_SIGNATURE" // SHA256(nonce + private key, certificate, timestamp)
});
otherIFrame.on("ready", function() {
// Style etc...
});
Only instantiate a new instance of the iFrame if you want to use multiple payment forms on the same host page. Otherwise, use the default
Spreedly
instance that is automatically created on the page.
Signature
SpreedlyPaymentFrame()
Set parameters
Sets parameters to bypass some validation features of the iframe. Valid parameters that can be passed are allow_blank_name
and allow_expired_date
. If set to true
, the related steps in validation will be skipped. Defaults to false
.
// On an active Spreedly instance
// Validation will not require any of the name fields
Spreedly.setParam('allow_blank_name', true);
// Validation will still require a date, but it can be expired
Spreedly.setParam('allow_expired_date', true);
Updated 7 days ago